The app itself consist of two button, one textview, one edit text and a spinner. The input in edit text is processed based on spinner value and the result is displayed on text edit. The button is just for checking and have fun, :)
That's it, now let's get our hand dirty...
Of course the first step is open AIDE apps, :)
then scroll down until "For Expert" choice appear, I didn't bother to use tutorial, you may like it though, :)
choose "Create new Project here", then choose "New App"
Fill the form
here my main.xml on res/layout
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
>
<TextView
android:id="@+id/tulisan"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:padding="10dp"
android:text="This is a simple two button app. \r\n \r\n Tell your user what your app does here \r\n \r\n \r\n \r\n"
android:textColor="#005500" />
<EditText
android:id="@+id/editText1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="10"
android:ems="10" >
<requestFocus />
</EditText>
<Spinner
android:id="@+id/sprOperator"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:entries="@array/sprSuhu"
/>
<Button
android:id="@+id/buttonStart"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Start"/>
<Button
android:id="@+id/buttonStop"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:text="Stop"/>
</LinearLayout>
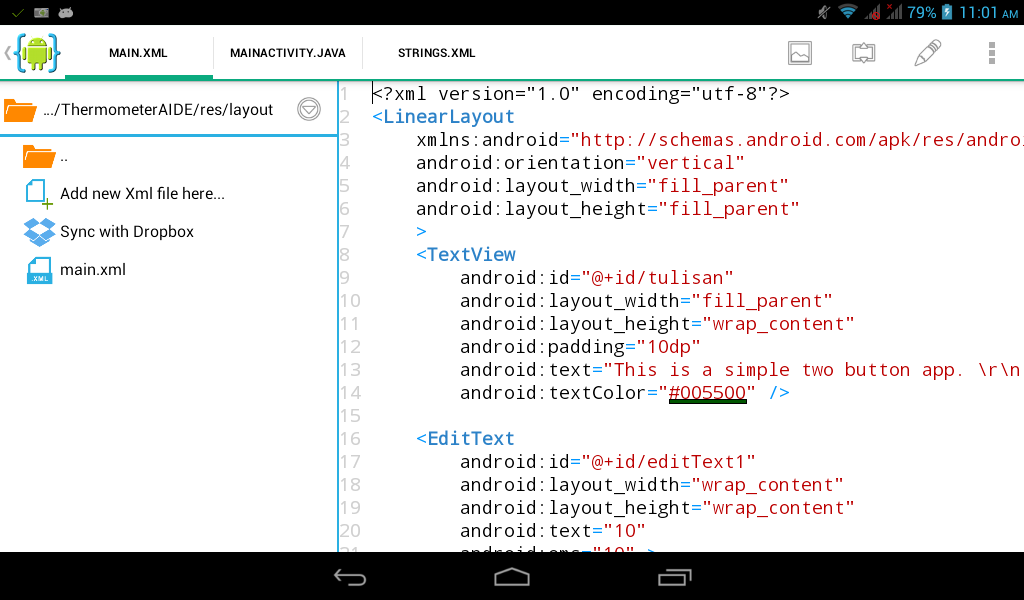
here my strings.xml on res/value
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">ThermometerPram</string>
<string name="hello_world">Hello world!</string>
<string name="action_settings">Settings</string>
<string-array name="sprSuhu">
<item >C</item>
<item >F</item>
<item >R</item>
<item >K</item>
</string-array>
</resources>
..and my MainActivity.java on src/com/nugnux/thermoaide
package com.nugnux.thermoaide;
import android.app.*;
import android.os.*;
import android.view.*;
import android.widget.*;
import android.view.View.OnClickListener;
public class MainActivity extends Activity
{
private static String logtag = "TwoButtonApp";//for use as the tag when logging
private static String text = "belum di pilih";//for use as the tag when logging
private static int pilihan = 0;//for use as the tag when logging
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
Spinner spinner = (Spinner) findViewById(R.id.sprOperator);
final String sprSuhu[] = getResources().getStringArray(R.array.sprSuhu);
ArrayAdapteradapter = ArrayAdapter.createFromResource(this,
R.array.sprSuhu, android.R.layout.simple_spinner_item);
spinner.setAdapter(adapter);
// Set the ClickListener for Spinner
spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
public void onItemSelected(AdapterView adapterView, View view, int i, long l) {
// TODO Auto-generated method stub
Toast.makeText(MainActivity.this,"Anda memilih satuan "
+ sprSuhu[i]+" ",Toast.LENGTH_SHORT).show();
text=sprSuhu[i];
pilihan=i;
hitung();
}
// If no option selected
public void onNothingSelected(AdapterView arg0) {
// TODO Auto-generated method stub
}
});
}
//Create an anonymous implementation of OnClickListener
private OnClickListener startListener = new OnClickListener() {
public void onClick(View v) {
//Log.d(logtag,"onClick() called - start button");
Toast.makeText(MainActivity.this, "The Start button was clicked.", Toast.LENGTH_LONG).show();
TextView tulisan = (TextView)findViewById(R.id.tulisan);
hitung();
//Log.d(logtag,"onClick() ended - start button");
}
};
// Create an anonymous implementation of OnClickListener
private OnClickListener stopListener = new OnClickListener() {
public void onClick(View v) {
//Log.d(logtag,"onClick() called - stop button");
Toast.makeText(MainActivity.this, "The Stop button was clicked.", Toast.LENGTH_LONG).show();
TextView tulisan = (TextView)findViewById(R.id.tulisan);
tulisan.setText("tadaa...");
//Log.d(logtag,"onClick() ended - stop button");
}
};
public void hitung(){
double hasil,suhu;
EditText editText1 =(EditText)findViewById(R.id.editText1);
TextView tulisan = (TextView)findViewById(R.id.tulisan);
tulisan.setText("hehehe, akhirnya...");
try {
hasil=Double.parseDouble(editText1.getText().toString());
tulisan.append(" \r\n(angka di bawah ini adalah "+Double.toString(hasil)+" )");
//hasil=Math.pow(hasil, 2);
tulisan.append(" \r\n[pangkatnya adalah "+Double.toString(Math.pow(hasil, 2))+" ] \r\nspinner bernilai "+text);
if (pilihan==0){
suhu=9./5.*hasil+32;
tulisan.append("\r\n Suhu "+Double.toString(hasil)+" C jika dikonversi akan menjadi bernilai:");
tulisan.append("\r\n"+Double.toString(suhu)+" F");
suhu=4./5.*hasil;
tulisan.append("\r\n"+Double.toString(suhu)+" R");
suhu=hasil+273;
tulisan.append("\r\n"+Double.toString(suhu)+" K");
}
if (pilihan==1){
suhu=5./9.*(hasil-32);
tulisan.append("\r\n Suhu "+Double.toString(hasil)+" F jika dikonversi akan menjadi bernilai:");
tulisan.append("\r\n"+Double.toString(suhu)+" C");
suhu=4./9.*(hasil-32);
tulisan.append("\r\n"+Double.toString(suhu)+" R");
suhu=(hasil-32)*5./9.+273;
tulisan.append("\r\n"+Double.toString(suhu)+" K");
}
if (pilihan==2){
suhu=9./4.*hasil+32;
tulisan.append("\r\n Suhu "+Double.toString(hasil)+" R jika dikonversi akan menjadi bernilai:");
tulisan.append("\r\n"+Double.toString(suhu)+" F");
suhu=5./4.*hasil;
tulisan.append("\r\n"+Double.toString(suhu)+" C");
suhu=hasil*5./4.+273;
tulisan.append("\r\n"+Double.toString(suhu)+" K");
}
if (pilihan==3){
suhu=9./5.*(hasil-273)+32;
tulisan.append("\r\n Suhu "+Double.toString(hasil)+" K jika dikonversi akan menjadi bernilai:");
tulisan.append("\r\n"+Double.toString(suhu)+" F");
suhu=4./5.*(hasil-273);
tulisan.append("\r\n"+Double.toString(suhu)+" R");
suhu=hasil-273;
tulisan.append("\r\n"+Double.toString(suhu)+" C");
}
} catch (NumberFormatException e) {
tulisan.setText(e.getMessage()+", masukkan angka");
}
}
}
run it

it will build
and prompt us whether we want to install the built app, of course...
here the result
No comments:
Post a Comment