from visual import *
from random import uniform
display(center=(0,2,0),background=(1,1,1), autoscale=False, range=4.5,
width=600, height=600, forward=(-.4,-.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
Ball = sphere(radius=2, length=4, opacity=.3)
bola = sphere(color=color.green,radius=.2)
bola.y = 1
bola.x = -1
bola.z = 1
v = vector(2,1,0)
dt = 1./16
r = bola.pos
rc = Ball.radius
def pantul():
global r,v
if mag(r)>=rc:
r = 1.99*norm(r)
vp = (dot(v,norm(r)))*norm(r)
vr = v-vp
v = vr - vp
def proses():
global r,v
a = vector(0,0,0)
v += a*dt
r += v*dt
bola.pos = r
pantul()
while 1:
rate(37)
proses()
Showing posts with label Python. Show all posts
Showing posts with label Python. Show all posts
Wednesday, May 25, 2016
Bouncing Ball inside Sphere
Tuesday, May 24, 2016
Bouncing inside Cylinder
from visual import *
from random import uniform
display(center=(0,2,0),background=(1,1,1), autoscale=False, range=4.5,
width=600, height=600, forward=(-.4,-.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
silinder = cylinder(radius=2, length=4, opacity=.3)
silinder.rotate(angle=pi/2, axis=(0,0,1),origin=(0,0,0))
bola = sphere(color=color.green,radius=.2)
bola.y = 0
bola.x = 0
bola.z = 1
v = vector(2,0,0)
dt = 1./16
r = bola.pos
rc = silinder.radius
def pantul():
global r,v
if mag(r)>=rc:
r = 1.9*norm(r)
vp = (dot(v,norm(r)))*norm(r)
vr = v-vp
v = vr - vp
def proses():
global r,v
a = vector(0,0,0)
v += a*dt
r += v*dt
bola.pos = r
pantul()
while 1:
rate(7)
proses()
Thursday, May 12, 2016
Bounce Over Spherical Surface
#code
from visual import * from random import uniform display(center=(0,2,0),background=(1,1,1), autoscale=False, range=7.5, width=600, height=600, forward=(-.4,-.3,-1)) #arah kamera distant_light(direction=(1,1,1), color=color.red) ball = sphere(radius=2, color=color.red, opacity = .5) r2 = ball.radius silinder = cylinder(radius=2, length=4, opacity=.3) silinder.rotate(angle=pi/2, axis=(0,0,1),origin=(0,0,0)) bola = sphere(color=color.green,radius=.2) bola.y = 3 bola.x = uniform(-1,1) bola.z = uniform(-1,1) v = vector(0,2,0) dt = 1./8. r = bola.pos def pantul(): global r,v print v if mag(r)<r2: print mag(r) arah = norm(r) dv = dot(v,arah) v -= dv*arah r = (r2+.2)*arah def proses(): global r,v a = vector(0,-1,0) v += a*dt r += v*dt bola.pos = r pantul() while 1: rate(11) proses()
Tuesday, May 10, 2016
N-Spring System
Using Visual Python
I like the result, :)
.
I like the result, :)
#code
from visual import *
n = 13
display(center=(n/2,0,0),background=(1,1,1), autoscale=False, range=(7),
width=600, height=600, forward=(-.4,-.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.orange)
dt = 1./8.
dx = []
k = []
m = []
l0 = []
l = []
v = []
x = []
for i in arange(n):
dx.append(.1)
k.append(1.)
m.append(1.)
l0.append(1.)
l.append(1.)
v.append(0.)
x.append(0.)
pegas = []
kotak = []
for i in arange(n):
spring = helix(pos=(0,0,0), axis=(5,0,0), radius=0.2, color=color.red, length=1.)
pegas.append(spring)
ko = box(pos=(0,0,0), width=.5, height=.5, length= .5, color= color.green)
kotak.append(ko)
box(pos=(-1,.64,0), width=n, height=2, length= 2, color=color.black)
box(pos=(n/2.,-.36,0), width=n, height=.2, length= n, color=color.white,opacity=.9)
#usikan
l[0] = 1.2
l[n-1] = .9
#posisi x
position = 0
for i in arange(n):
position +=l[i]
x[i] = position
kotak[i].x = x[i]
def updatePegas():
global l
for i in arange(n):
if i!=0:
pegas[i].x = x[i-1]
l[i] = x[i]-x[i-1]
else:
l[i] = x[i]
kotak[i].x = x[i]
pegas[i].length = l[i]
def proses():
for i in arange(n):
dx[i] = l[i]-l0[i]
f0 = -k[i]*dx[i]
if i<n-1:
dx[i+1] = l[i+1]-l0[i+1]
f1 = -k[i+1]*dx[i+1]
a = (f0-f1)/m[i]
else:
a = f0/m[i]
v[i] += a*dt
x[i] += v[i]*dt
updatePegas()
while 1:
rate (19)
proses()
Monday, May 9, 2016
Here's the Culprit
In Visual Python, helix object will generate error if helix.length = some array like the code below. I use dl, an array, for the length value
from visual import *
display(center=(1,0,0),background=(1,1,1), autoscale=False, range=(2,2,2),
width=600, height=600, forward=(-.4,-.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
n = 1
dt = 1./8.
dl = ones(1)
pegas = []
print dl
for i in arange(n):
spring = helix(pos=(0,0,0), axis=(5,0,0), radius=0.2, color=color.red, length=1.)
pegas.append(spring)
def updatePegas(l):
pegas[0].length = l
def proses():
global dl
l = pegas[0].length
if l>2:
l = 2
dl[0] *= -1
elif l<.5:
l = .5
dl[0] *= -1
l += dl[0]
updatePegas(l)
while 1:
rate (19)
proses()
And the result is
Traceback (most recent call last):
File "springList.py", line 40, in
proses()
File "springList.py", line 36, in proses
updatePegas(l)
File "springList.py", line 22, in updatePegas
pegas[0].length = l
File "/Library/Frameworks/Python.framework/Versions/2.7/lib/python2.7/site-packages/VPython-6.11-py2.7-macosx-10.6-intel.egg/visual_common/primitives.py", line 850, in set_length
self.__frame.axis = self.__axis.norm()
AttributeError: 'numpy.ndarray' object has no attribute 'norm'
If we change dl from array to list, like the code below, everything is suddenly OK, :)
from visual import *
display(center=(1,0,0),background=(1,1,1), autoscale=False, range=(2,2,2),
width=600, height=600, forward=(-.4,-.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
n = 1
dt = 1./8.
#dl = ones(1)
dl = []
dl.append(.1)
pegas = []
print dl
for i in arange(n):
spring = helix(pos=(0,0,0), axis=(5,0,0), radius=0.2, color=color.red, length=1.)
pegas.append(spring)
def updatePegas(l):
pegas[0].length = l
def proses():
global dl
l = pegas[0].length
if l>2:
l = 2
dl[0] *= -1
elif l<.5:
l = .5
dl[0] *= -1
l += dl[0]
updatePegas(l)
while 1:
rate (19)
proses()
Gonna rewrite the code.
Sunday, May 8, 2016
Double Spring System
Akhirnya.
With Visual Python module
I couldn't use list for spring length since it'll trigger some error for helix object. It's very unfortunate because it would come handy as we expand the number of spring and mass.
Anyway, here's the code
.
With Visual Python module
I couldn't use list for spring length since it'll trigger some error for helix object. It's very unfortunate because it would come handy as we expand the number of spring and mass.
Anyway, here's the code
#code
from visual import *
from random import uniform,random
from visual.controls import *
display(center=(1,0,0),background=(1,1,1), autoscale=False, range=(2,2,2),
width=600, height=600, forward=(-.4,-.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
n = 2
dt = 1./8.
pegas = []
kotak = []
box(pos=(-1,0,0), width=2, height=2, length= 2, color=color.black)
box(pos=(0,-.36,0), width=2, height=.2, length= 5, color=color.black,opacity=.3)
for i in arange(n):
spring = helix(pos=(0,0,0), axis=(5,0,0), radius=0.2, color=color.red, length=1.)
pegas.append(spring)
ko = box(pos=(0,0,0), width=.5, height=.5, length= .5, color= color.green)
kotak.append(ko)
k0 = 1.
k1 = 1.
m0 = 1.
m1 = 1.
l00 = 1.
l01 = 1.
l0 = 1.
l1 = 1.1
x0 = l0
x1 = l0+l1
v0 = 0.
v1 = 0.
y = 1.
print x1
def updatePegas():
global x0,x1
kotak[0].x = x0
pegas[0].length = l0
kotak[1].x = x1
pegas[1].x = l0
pegas[1].length = l1
def proses():
global l0,v0,x0,l1,v1,x1
#untuk m0
dx0 = l0-l00
f0 = -k0*dx0
dx1 = l1-l01
f1 = -k1*dx1
a0 = (f0-f1)/m0
v0 += a0*dt
x0 += v0*dt
l0 = x0
#untuk m1
a1 = f1/m1
v1 += a1*dt
x1 += v1*dt
l1 = x1-x0
updatePegas()
while 1:
rate (39)
y += .1
proses()
Saturday, May 7, 2016
Normal Mode
Planned to write coupled oscillator, using double spring.
For some reason, Vpython refused to set length of helix with some value from array or list or any value derived from them. It only accept plain number or number from simple variable (like a = 3. ). It hugely messed the whole script as I used l = [] for helix length.
So, rewriting the code, create l0 and l1 manually.
Didn't have energy to code the rest. So, at the moment, just call it "normal mode" coupled oscillator, heheh...
#code
from visual import *
from random import uniform,random
from visual.controls import *
display(center=(0,0,0),background=(1,1,1), autoscale=False, range=(5,5,3),
width=600, height=600, forward=(-1.4,-1.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
n = 2
dt = 1./8.
pegas = []
kotak = []
w = ones(n)
w /= 2.
for i in arange(n):
spring = helix(pos=(0,0,0), axis=(5,0,0), radius=0.2, color=color.red, length=1.)
pegas.append(spring)
ko = box(pos=(0,0,0), width=.5, height=.5, length= .5, color= color.green)
kotak.append(ko)
k0 = 1.
m0 = 1.
l00 = 1.
l01 = 1.
l0 = 1.1
l1 = 1.
x0 = l0+w[0]/2
v0 = 0.
v1 = 0.
y = 1.
print pegas[0].length
def updatePegas():
global x0
kotak[0].x = x0
pegas[0].length = l0
x1 = l0+w[0]+l1+w[1]/2
kotak[1].x = x1
pegas[1].x = l0+w[0]
def proses():
global l0,v0,x0
dx = l0-l00
f0 = -k0*dx
a0 = f0/m0
v0 += a0*dt
l0 += v0*dt
x0 = l0+w[0]/2
updatePegas()
while 1:
rate (19)
y += .1
proses()
Friday, May 6, 2016
Spring.
Bahan baru buat ide jahil besok, :)
.
#code
from visual import *
from random import uniform,random
from visual.controls import *
display(center=(0,0,0),background=(1,1,1), autoscale=False, range=(3,5,3),
width=600, height=600, forward=(-1.4,-1.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
spring = helix(pos=(0,2,1), axis=(5,0,0), radius=0.5, color=color.red)
dl = .1
l = 1.
def proses():
global l,dl
spring.length = l
if l>2:
l = 2
dl *= -1
elif l<.5:
l = .5
dl *= -1
l += dl
while 1:
rate (19)
proses()
Piston Free Expansion Oscillation.
The result of daydreaming, :)
.
#code
from visual import *
from random import uniform,random
from visual.controls import *
display(center=(0,0,0),background=(1,1,1), autoscale=False, range=(5,7,7),
width=600, height=600, forward=(0.4,-0.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
cylinder(pos=(-4,0,0), axis=(7,0,0), radius=1, color=color.green, opacity=.1)
piston = cylinder(pos=(-2,0,0), axis=(1,0,0), radius=.7, color=color.red)
piston1 = cylinder(pos=(-2,0,0), axis=(5,0,0), radius=.1, color=color.red)
p0 = 1.
A = 1.
x0 = 5. #panjang piston
c = p0*A*x0
x = 3
dx = x0-x #
m = 1.
v = 0.
dt = 1./8.
def proses():
global x,v,dx
p = c/x
dp = p-p0
print x,dp
f = -dp
a = f/m
v += a*dt
dx += v*dt
x -= v*dt
piston.x = dx
piston1.x = dx
while 1:
rate (19)
proses()
Ball Oscillation Inside a Sphere
with Visual Python.
I use nonlinear solution, :)
.
I use nonlinear solution, :)
#code
from visual import *
from random import uniform,random
from visual.controls import *
display(center=(0,0,0),background=(1,1,1), #autoscale=False,
width=600, height=600, forward=(-0.4,-0.3,-1)) #arah kamera
distant_light(direction=(1,1,1), color=color.red)
dl = .01
l = 1.1
box(color=color.white, pos=(0,0,0),length=2*l,height=dl, width=dl, opacity=.3)
box(color=color.white, pos=(0,0,0),length=dl,height=2*l, width=dl, opacity=.3)
box(color=color.white, pos=(0,0,0),length=dl,height=dl, width=2*l, opacity=.3)
bola1 = sphere (pos=(0,0,0), radius=1.1, color=color.green, opacity=.3)
bola = sphere (pos=(1,0,0), radius=.1, color=color.red)
r = 1.
x = 1.
m = 1.
g = 1.
vx = 0.
dt = 1./16.
def proses():
global x,vx
f = -m*g*x/r
a = f/m
vx += a*dt
x += vx*dt
r2 = r*r
x2 = x*x
if x2>r2:
x2 = r2
y = r-sqrt(r2-x2)
bola.x = x
print x
bola.y = y-r
while 1:
rate (19)
proses()
Thursday, April 28, 2016
3D Ball Collision in Python with Visual Module.
I only compute the collision between bed and blue one. They're bola[1] and bola[2] respectively
from visual import *
from random import uniform,random
from visual.controls import *
def change():
global jalan
if b.value:
jalan = True
for ball in(bola):
ball.vx = uniform(-7,7)
ball.vy = uniform(-7,7)
ball.vz = uniform(-7,7)
else:
jalan = False
c = controls(title='Tempat Tombol',x=800, y=0, width=300, height=300, range=50)
b = toggle( pos=(0,0), width=20, height=20, text='Click me', action=lambda: change() )
display(center=(0,0,0),background=(1,1,1), #autoscale=False,
width=600, height=600, forward=(-0.4,-0.3,-1)) #arah kamera
g = -1.
dt = .1
e = 1.
b.value = True
jalan = True
l = 17.
dl = .01
n = 11
distant_light(direction=(1,1,1), color=color.red)
lantai = box(color=color.white, pos=(0,0,0),length=l,height=dl, width=l, opacity=.3)
dindingKiri = box(color=color.white, pos=(-l/2,l/2,0),length=dl,height=l, width=l, opacity=.3)
dindingKanan= box(color=color.white, pos=(l/2,l/2,0),length=dl,height=l, width=l, opacity=.3)
dBelakang = box(color=color.white, pos=(0,l/2,-l/2),length=l,height=l, width=dl, opacity=.3)
atap = box(color=color.white, pos=(0,l,0),length=l,height=dl, width=l, opacity=.3)
bola = []
for i in arange(n):
ball = sphere (pos=(uniform(1,7),0,uniform(-7,7)), radius=.3, color=color.green)
ball.v = vector(uniform(-7,7),uniform(-7,7),uniform(-7,7))
bola.append(ball)
bola[1].radius = 2.5
bola[2].radius = 2.5
bola[1].color = color.red
bola[2].color = color.blue
def proses():
for ball in (bola):
a = g
ball.v[1] += a*dt
ball.pos+= ball.v*dt
tumbukan()
tumbukanBola()
def tumbukan():
for ball in(bola):
if ball.y<0:
ball.y = 0.01
ball.v[1] *=-1.*e
elif ball.y>l:
ball.y = l-.01
ball.v[1] *= -1
if ball.x<-l/2:
ball.x=-l/2+.01
ball.v[0] *= -1*e
if ball.x>l/2:
ball.x=l/2-.01
ball.v[0] *= -1*e
if ball.z<-l/2:
ball.z=-l/2+.01
ball.v[2] *= -1*e
if ball.z>l/2:
ball.z=l/2-.01
ball.v[2] *= -1*e
def tumbukanBola():
#pass
jarak = mag(bola[2].pos-bola[1].pos)
if jarak<(bola[1].radius+bola[2].radius):
arah = norm(bola[2].pos-bola[1].pos)
v1 = dot(bola[1].v,arah)
v2 = dot(bola[2].v,arah)
dv = v2-v1
bola[1].v += dv*arah
bola[2].v -= dv*arah
while 1:
rate (51)
if jalan:
proses()
Alright, Now Do It in Python, with style, :)
Python version of this flash action script of electron under Lorentz force, :)
from visual import *
from random import uniform
display(center=(0,0,0),background=(1,1,1), autoscale=False,
width=600, height=600,
#forward=(-0.4,-0.3,-1)
)
distant_light(direction=(1,1,1), color=color.red)
l = 11
dt = 1./8.
medan = box(color=color.white,
pos=(l/2,0,0),length=l,height=l,
width=l, opacity=.3)
ball = sphere (pos=(-7,0,0), radius=.3, color=(uniform(0,1),uniform(0,1),uniform(0,1)))
def awal():
global q,m,B,v
q = 1.
m = 1.
B = vector(0.,0.,1.)
v = vector(3.,0.,0.)
def proses():
global v,B
if ball.x > 0:
B = vector(0.,0.,1.)
else:
B = vector(0.,0.,0.)
print B
F = q*(cross(v,B))
a = F/m
v += a*dt
ball.pos += v*dt
print ball.x
awal()
while 1:
rate (37)
proses()
Wednesday, April 27, 2016
Iterasi di Python
Jika kita punya sebuah list bernama bola yang didefinisikan sebagai:
bola = []
kemudian kita membuat obyek bernama ball
ball = sphere (pos=(uniform(1,7),0,uniform(-7,7)), radius=.3, color (uniform(0,1),uniform(0,1),uniform(0,1)))
ball.vx = uniform(-7,7)
ball.vy = uniform(-7,7)
ball.vz = uniform(-7,7)
Obyek ini kita masukkan ke dalam bola dengan perintah
bola.append(ball)
Kita dapat melakukannya berkali-kali sehingga pada list bola terdapat beberapa obyek bernama ball
Jika kita ingin mengakses obyek tersebut, kita dapat menggunakan perintah semacam
bola[0].vx = 1
jika kita ingin mengakses vx di semua obyek bola, kita dapat menggunakan iterasi
for i in arange (n):
bola[i].vx = 1
Namun di python ada cara lain yang juga mudah
for ball in (bola):
ball.vx = 1
Berikut contoh kode yang menggunakan iterasi seperti itu
from visual import *
from random import uniform,random
from visual.controls import *
def change():
global jalan,vx,vy,vz
if b.value:
jalan = True
for ball in(bola):
ball.vx = uniform(-7,7)
ball.vy = uniform(-7,7)
ball.vz = uniform(-7,7)
else:
jalan = False
c = controls(title='Tempat Tombol',x=800, y=0, width=300, height=300, range=50)
b = toggle( pos=(0,0), width=20, height=20, text='Click me', action=lambda: change() )
display(center=(0,0,0),background=(1,1,1), #autoscale=False,
width=600, height=600, forward=(-0.4,-0.3,-1)) #arah kamera
g = -1.
dt = .1
e = 1.
b.value = True
jalan = True
l = 17.
dl = .01
n = 11
distant_light(direction=(1,1,1), color=color.red)
lantai = box(color=color.white, pos=(0,0,0),length=l,height=dl, width=l, opacity=.3)
dindingKiri = box(color=color.white, pos=(-l/2,l/2,0),length=dl,height=l, width=l, opacity=.3)
dindingKanan= box(color=color.white, pos=(l/2,l/2,0),length=dl,height=l, width=l, opacity=.3)
dBelakang = box(color=color.white, pos=(0,l/2,-l/2),length=l,height=l, width=dl, opacity=.3)
atap = box(color=color.white, pos=(0,l,0),length=l,height=dl, width=l, opacity=.3)
bola = []
for i in arange(n):
ball = sphere (pos=(uniform(1,7),0,uniform(-7,7)), radius=.3, color=(uniform(0,1),uniform(0,1),uniform(0,1)))
ball.vx = uniform(-7,7)
ball.vy = uniform(-7,7)
ball.vz = uniform(-7,7)
bola.append(ball)
def proses():
global vx,vy,vz
for ball in (bola):
a = g
ball.vy += a*dt
ball.pos+= vector(ball.vx*dt,ball.vy*dt,ball.vz*dt)
tumbukan()
def tumbukan():
global vx,vy,vz
for ball in(bola):
if ball.y<0:
ball.y = 0.01
ball.vy *=-1.*e
elif ball.y>l:
ball.y = l-.01
ball.vy *= -1
if ball.x<-l/2:
ball.x=-l/2+.01
ball.vx *= -1*e
if ball.x>l/2:
ball.x=l/2-.01
ball.vx *= -1*e
if ball.z<-l/2:
ball.z=-l/2+.01
ball.vz *= -1*e
if ball.z>l/2:
ball.z=l/2-.01
ball.vz *= -1*e
while 1:
rate (100)
if jalan:
proses()
Thursday, April 21, 2016
Lorenz Attractor in Python with Visual Module.
So much faster than matplotlib 3d projection
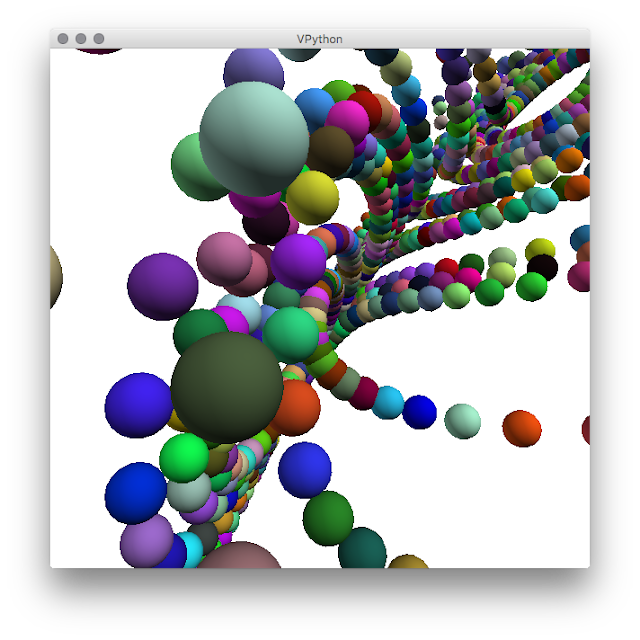
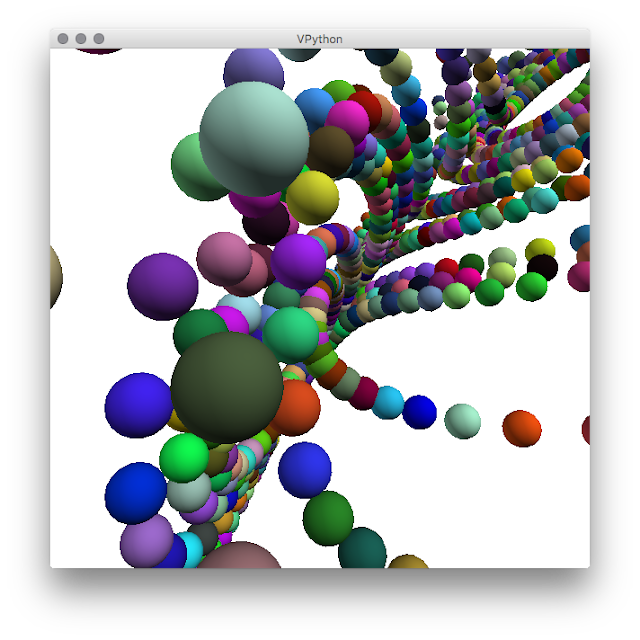
from visual import *
from random import uniform
display(center=(0,0,0), #pusat display
background=(1,1,1),
#autoscale=False, #agar display tidak otomatis mengikuti obyek
width=600,
height=600,
forward=(-0.4,-0.3,-1)) #arah kamera
x = 1.
y = 1.
z = 1.
dt = 1./64.
s = 10.
b = 8./3.
r = 28
w = 0.
dw = 0.01
n = 0
while 1:
rate(1)
while n<3000:
n += 1
xdot = s * (y-x)
ydot = x*r -x*z -y
zdot = x*y -b*z
x = x+xdot*dt
y = y+ydot*dt
z = z+zdot*dt
w +=dw
if w>1 or w<0:
dw = -dw
sphere(pos=(x,y,z),radius=.7,color=(0.,w,0))
Saturday, April 16, 2016
Forced to get Force using Center of Mass
I know. Using solely center of mass as main contributor in force calculation is bad idea in nearly homogenous n-body system.
Well, I'm curious about that. So, just bite it.
And, yeah, bad idea.
The system is fine, generally.
Each body move as usual, at a glimpse.
But if we go detail about that. There's strange behavior here and there.
On previous code with brute force calculation, we could saw, binary system consist of two body orbiting each other. But, with this center-of-mass-code, we see no one. A body that have very close neighbor don't make a twin system. It continues orbiting center of mass.
As we have system with -body with same mass, it should be natural if two close-body will have greater gravity force than with any other body. It should have "priority"; orbiting each other.
Ok, this "forced"-center-mass-code is weird, but it'll useful in other system. :)
.
Well, I'm curious about that. So, just bite it.
And, yeah, bad idea.
The system is fine, generally.
Each body move as usual, at a glimpse.
But if we go detail about that. There's strange behavior here and there.
On previous code with brute force calculation, we could saw, binary system consist of two body orbiting each other. But, with this center-of-mass-code, we see no one. A body that have very close neighbor don't make a twin system. It continues orbiting center of mass.
As we have system with -body with same mass, it should be natural if two close-body will have greater gravity force than with any other body. It should have "priority"; orbiting each other.
Ok, this "forced"-center-mass-code is weird, but it'll useful in other system. :)
from visual import *
from random import uniform,random
l = 17.
dl = .01
display(center=(0,0,0),background=(1,1,1),autoscale=False, width=600, height=600,forward=(-0.7,-0.7,-1))
distant_light(direction=(1,1,1), color=color.red)
#local_light(pos=(0,0,0), color=(0,0,1))
#sphere(pos=(0,0,0), color=(0,0,0), material=materials.emissive, opacity=0.9)
box(color=color.white, pos=(0,l/2,0),length=l,height=dl, width=l, opacity=0.3)
box(color=color.white, pos=(0,-l/2,0),length=l,height=dl, width=l, opacity=0.3)
box(color=color.white, pos=(l/2,0,0),length=dl,height=l, width=l, opacity=0.3)
box(color=color.white, pos=(-l/2,0,0),length=dl,height=l, width=l, opacity=0.3)
box(color=color.white, pos=(0,0,l/2),length=l,height=l, width=dl, opacity=0.3)
bola = []
n = 11
dv = .1 #kecepatan setelah menabrak dinding
G = 31.
for i in range(n):
ball = sphere (pos=(uniform(-7,7),uniform(-7,7),uniform(-7,7)), radius=.2, color=(random(),random(),random()))
ball.v = vector(uniform(-1,1),uniform(-1,1),uniform(-1,1))
bola.append(ball)
dt = 1./32.
bola[0].pos=(1,1,1)
def hitungGaya(i,rcm):
r = bola[i].pos-rcm
jarak = mag(r)
arah = -norm(r)
gaya = G*1./(pow(jarak,2)+.1)*arah*n
return gaya
def hitungPusatMassa():
xcm = 0.
ycm = 0.
zcm = 0.
for i in arange(n):
xcm += bola[i].x
ycm += bola[i].y
zcm += bola[i].z
xcm /= n
ycm /= n
zcm /= n
return vector(xcm,ycm,zcm)
def proses():
global bola
rcm = hitungPusatMassa()
for i in arange(n):
gaya = hitungGaya(i,rcm)
r = bola[i].pos
#tambah gaya dari pusat
'''
jarak = mag(bola[i].pos)
arah = -norm(bola[i].pos)
gaya += 10*G*1./(pow(jarak,2)+.05)*arah
'''
a = gaya
v = bola[i].v
v += a*dt
r += v*dt
#cek pantul ke tembok
if bola[i].x > l/2.:
bola[i].x = l/2.
v.x *= -dv
elif bola[i].x < -l/2.:
bola[i].x = -l/2.
v.x *= -dv
elif bola[i].y > l/2.:
bola[i].y = l/2.
v.y *= -dv
if bola[i].y < -l/2.:
bola[i].y = -l/2.
v.y *= -dv
if bola[i].z > l/2.:
bola[i].z = l/2.
v.z *= -dv
if bola[i].z < -l/2.:
bola[i].z = -l/2.
v.z *= -dv
bola[i].pos = r
while 1:
rate (10)
proses()
Menjinakkan Gravitasi.
Cari cara agar mereka jadi enak untuk dilihat.
Di kode sebelumnya, bola sudah dicegah agar tidak melarikan diri dengan menambahkan kotak, mereka akan memantul jika menabrak kotak.
Beres, sekarang tambahkan interaksi antar bola, interaksi sederhana, gravitasi.
Ok, pake metode brutal, untuk tiap-tiap bola, hitung satu-satu interaksi gravitasi dengan seluruh bola, kemudian jumlahkan.

(ide, cari pusat masa seluruh bola, perlakukan interaksi gaya seperti kode sebelumnya)
(saat nulis tadi, sambil mikir, ternyata itu ide buruk, tapi boleh juga, lihat saja nanti, bagus atau tidak)
(tapi tidak sekarang, tidak masuk kode yang sekarang)
(kode di posting ini tetap pake metode brutal)
Hasilnya adalah, ..., ok, mereka tetap memantul saat menabrak tembok, tetapi di dalam kotak mereka bergerak dengan sangat cepat, bola liar.
(yep, metode brutal menghasilkan hasil brutal, tetapi tidak selalu...)
Ide licik, tambahkan koefisien restitusi di dinding, tumbukan dengan dinding tidak lenting sempurna, kecepatannya tinggal 10% setelah memantul
Dengan demikian mereka lebih jinak di dalam kotak, :)
from visual import *
from random import uniform,random
l = 17.
dl = .01
display(center=(0,0,0),background=(1,1,1),autoscale=False, width=600, height=600,forward=(-0.7,-0.7,-1))
distant_light(direction=(1,1,1), color=color.red)
#local_light(pos=(0,0,0), color=(0,0,1))
#sphere(pos=(0,0,0), color=(0,0,0), material=materials.emissive, opacity=0.9)
box(color=color.white, pos=(0,l/2,0),length=l,height=dl, width=l, opacity=0.3)
box(color=color.white, pos=(0,-l/2,0),length=l,height=dl, width=l, opacity=0.3)
box(color=color.white, pos=(l/2,0,0),length=dl,height=l, width=l, opacity=0.3)
box(color=color.white, pos=(-l/2,0,0),length=dl,height=l, width=l, opacity=0.3)
box(color=color.white, pos=(0,0,l/2),length=l,height=l, width=dl, opacity=0.3)
bola = []
n = 11
dv = .1 #kecepatan setelah menabrak dinding
G = 31.
for i in range(n):
ball = sphere (pos=(uniform(-7,7),uniform(-7,7),uniform(-7,7)), radius=.3, color=(random(),random(),random()))
ball.v = vector(uniform(-1,1),uniform(-1,1),uniform(-1,1))
bola.append(ball)
dt = 1./32.
bola[0].pos=(1,1,1)
def hitungGaya(i):
gaya = vector(0,0,0)
for j in arange(n):
if i!=j:
r = bola[i].pos-bola[j].pos
jarak = mag(r)
arah = -norm(r)
gaya += G*1./(pow(jarak,2)+.1)*arah
return gaya
def proses():
global bola
for i in arange(n):
gaya = hitungGaya(i)
#tambah gaya dari pusat
r = bola[i].pos
jarak = mag(bola[i].pos)
arah = -norm(bola[i].pos)
gaya += 10*G*1./(pow(jarak,2)+.05)*arah
a = gaya
v = bola[i].v
v += a*dt
r += v*dt
#cek pantul ke tembok
if bola[i].x > l/2.:
bola[i].x = l/2.
v.x *= -dv
elif bola[i].x < -l/2.:
bola[i].x = -l/2.
v.x *= -dv
elif bola[i].y > l/2.:
bola[i].y = l/2.
v.y *= -dv
if bola[i].y < -l/2.:
bola[i].y = -l/2.
v.y *= -dv
if bola[i].z > l/2.:
bola[i].z = l/2.
v.z *= -dv
if bola[i].z < -l/2.:
bola[i].z = -l/2.
v.z *= -dv
bola[i].pos = r
while 1:
rate (10)
proses()
Friday, April 15, 2016
Bermain dengan Gaya Sentral di Python.
Merangkak dari kode sebelumnya, dengan tambahan kotak yang mengungkung bola-bola yang mengitari titik asal dengan berbagai kecepatan. Bola-bola tersebut tak bisa menembus kotak, jika menabrak salah satu dinding kotak, maka dia akan memantul
from visual import *
from random import uniform,random
l = 17.
dl = .01
display(center=(0,0,0),background=(1,1,1),autoscale=False, width=600, height=600,forward=(-0.7,-0.7,-1))
distant_light(direction=(1,1,1), color=color.red)
box(color=color.white, pos=(0,l/2,0),length=l,height=dl, width=l, opacity=0.3)
box(color=color.white, pos=(0,-l/2,0),length=l,height=dl, width=l, opacity=0.3)
box(color=color.white, pos=(l/2,0,0),length=dl,height=l, width=l, opacity=0.3)
box(color=color.white, pos=(-l/2,0,0),length=dl,height=l, width=l, opacity=0.3)
box(color=color.white, pos=(0,0,l/2),length=l,height=l, width=dl, opacity=0.3)
bola = []
n = 11
G = 31.
for i in range(n):
ball = sphere (pos=(uniform(1,7),0,uniform(-7,7)), radius=.3, color=(random(),random(),random()))
ball.v = vector(0,uniform(1,3),0)
bola.append(ball)
dt = 1./32.
bola[0].pos=(1,1,1)
while 1:
rate (100)
for i in arange(n):
r = bola[i].pos
v = bola[i].v
jarak = mag(bola[i].pos)
arah = -norm(bola[i].pos)
gaya = G*1./(pow(jarak,2)+.1)*arah
a = gaya
v += a*dt
r += v*dt
#cek pantul ke tembok
if bola[i].x > l/2.:
bola[i].x = l/2.
v.x *= -1
elif bola[i].x < -l/2.:
bola[i].x = -l/2.
v.x *= -1
elif bola[i].y > l/2.:
bola[i].y = l/2.
v.y *= -1
if bola[i].y < -l/2.:
bola[i].y = -l/2.
v.y *= -1
if bola[i].z > l/2.:
bola[i].z = l/2.
v.z *= -1
if bola[i].z < -l/2.:
bola[i].z = -l/2.
v.z *= -1
bola[i].pos = r
Thursday, April 14, 2016
Playing with Central Force in Pyton with Visual Module
I don't use n body calculation, every sphere only attracted by force from origin, dependent upon its position.
from visual import *
from random import uniform,random
l = 17.
dl = .01
display(center=(0,0,0),background=(1,1,1),autoscale=False, width=600, height=600,forward=(-0.4,-0.3,-1))
distant_light(direction=(1,1,1), color=color.red)
box(color=color.white, pos=(0,0,0),length=l,height=dl, width=l, opacity=0.3)
box(color=color.white, pos=(0,0,0),length=dl,height=l, width=l, opacity=0.3)
box(color=color.white, pos=(0,0,0),length=l,height=l, width=dl, opacity=0.3)
bola = []
n = 11
G = 31.
for i in range(n):
ball = sphere (pos=(uniform(1,7),0,uniform(-7,7)), radius=.3, color=(random(),random(),random()))
ball.v = vector(0,uniform(1,3),0)
bola.append(ball)
dt = 1./32.
bola[0].pos=(1,1,1)
while 1:
rate (100)
for i in arange(n):
r = bola[i].pos
v = bola[i].v
jarak = mag(bola[i].pos)
arah = -norm(bola[i].pos)
gaya = G*1./(pow(jarak,2)+.1)*arah
a = gaya
v += a*dt
r += v*dt
bola[i].pos = r
Wave Simulation Using Visual Python.
I used previous code (with matplotlib animation).
Removed the matplotlib part, swap it with vpython, :)
"""
Gelombang
"""
import numpy as np #untuk operasi array
from visual import *
#variabel
n = 39
x = np.arange(0., 1.,1./n ) #array dari 0 s.d 1 berjarak 1/n
y = np.zeros(n) #array sejumlah n isinya 0
y1 = np.zeros(n) # y1 : array [0..n] of real
y2 = np.zeros(n)
y1 = np.exp(-1*np.power(10*x-3,2))
y2 = np.exp(-1*np.power(10*x-3,2))
r2 = 1./512
display(center=(.5,0,0),background=(1,1,1))
#kotak
dindingKiri = box (pos=(0.,0,0), length=.01, height=1, width=1, color=color.green)
dindingKanan = box (pos=(1.,0,0), length=.01, height=1, width=1, color=color.blue)
bola = []
for i in range(n):
ball = sphere (pos=(x[i],y2[i],0), radius=.01, color=color.red)
bola.append(ball)
#nilai awal fungsi gaussian
#print y2
def proses():
#hitung nilai baru
for i in np.arange(1,n-1):
y[i] = 2*(1-r2)*y1[i]-y2[i]+r2*(y1[i+1]+y1[i-1])
#geser
y2[:] = y1[:]
y1[:] = y[:]
#print y2
return y2
while 1:
rate(100)
proses()
for i in np.arange(n):
bola[i].y = y2[i]


Wednesday, April 13, 2016
Hello (Again) Visual Python, :D .
Menyapa kembali mainan lama, :)
from visual import *
floor = box (pos=(0,0,0), length=4, height=0.5, width=4, color=color.blue)
bola = []
n = 2
for i in range(n):
ball = sphere (pos=(0,4,0), radius=1, color=color.red)
ball.v = vector(0,-1,0)
bola.append(ball)
dt = 0.01
bola[1].pos=(1,1,1)
while 1:
rate (100)
bola[0].pos = bola[0].pos + bola[0].v*dt
if bola[0].y < bola[0].radius:
bola[0].v.y = abs(bola[0].v.y)
else:
bola[0].v.y = bola[0].v.y - 9.8*dt

Subscribe to:
Posts (Atom)
My sky is high, blue, bright and silent.
Nugroho's (almost like junk) blog
By: Nugroho Adi Pramono
323f
(5)
amp
(1)
android
(12)
apple
(7)
arduino
(18)
art
(1)
assembler
(21)
astina
(4)
ATTiny
(23)
blackberry
(4)
camera
(3)
canon
(2)
cerita
(2)
computer
(106)
crazyness
(11)
debian
(1)
delphi
(39)
diary
(286)
flash
(8)
fortran
(6)
freebsd
(6)
google apps script
(8)
guitar
(2)
HTML5
(10)
IFTTT
(7)
Instagram
(7)
internet
(12)
iOS
(5)
iPad
(6)
iPhone
(5)
java
(1)
javascript
(1)
keynote
(2)
LaTeX
(6)
lazarus
(1)
linux
(29)
lion
(15)
mac
(28)
macbook air
(8)
macbook pro
(3)
macOS
(1)
Math
(3)
mathematica
(1)
maverick
(6)
mazda
(4)
microcontroler
(35)
mountain lion
(2)
music
(37)
netbook
(1)
nugnux
(6)
os x
(36)
php
(1)
Physicist
(29)
Picture
(3)
programming
(189)
Python
(109)
S2
(13)
software
(7)
Soliloquy
(125)
Ubuntu
(5)
unix
(4)
Video
(8)
wayang
(3)
yosemite
(3)